deepseek r1
https://console.groq.com/playground?model=deepseek-r1-distill-llama-70b
Me:
Make a web based rpg game, top down view, pixel graphics, very very minimal, one should be able to walk with wasd and destroy trees to pick up wood. any optimization allowed. we also need a win condition and text saying something appropriate. Also a countdown timer, and text showing if we lost the game.
Deepseek:
<think>
Okay, so the user wants to create a web-based RPG game with specific features. Let me break down the requirements to understand what needs to be done.
First, the game should be top-down view with pixel graphics. That means using a 2D canvas or a library that handles 2D rendering. Since the user mentioned minimalism, I should keep the graphics simple, maybe using basic shapes and colors.
The player should be able to move with WASD keys. So, I'll need to set up event listeners for key presses and update the player's position accordingly. The player's sprite can be a simple shape like a circle or a square.
Next, the ability to destroy trees to collect wood. Trees can be represented as static objects on the map. When the player interacts with a tree (maybe by pressing 'E'), the tree disappears, and the player's wood count increases. I'll need to handle collision detection between the player and trees.
A win condition is needed. The user didn't specify what the win condition is, so I'll have to define it. Maybe collecting a certain amount of wood, like 10 pieces, triggers the win. Once the player collects enough wood, display a win message.
There should also be a countdown timer. The game should last for a certain duration, say 30 seconds. If the timer runs out before the player collects enough wood, the player loses. I'll need to set up a timer that counts down each second and check if it reaches zero.
Text displays are necessary to show the wood count, timer, and win/lose messages. Using the canvas, I can draw text for these elements. The win message could say "You Win!" and the lose message "You Lose!".
Optimization is allowed, so I'll make sure the game runs smoothly. Using HTML5 Canvas is efficient for this purpose. Keeping the graphics minimal will help with performance.
Putting it all together, I'll structure the game loop to handle drawing, updating positions, checking collisions, and managing the timer. Event listeners will handle user input for movement and interacting with trees.
I'll start by setting up the HTML structure with a canvas and necessary styles. Then, in the JavaScript, define the player, trees, and game mechanics. The game loop will update the game state, check for wins or losses, and render everything on the canvas.
Testing each feature as I go will ensure everything works. For example, moving the player with WASD, collecting wood by pressing 'E' near a tree, and checking the win condition when wood reaches 10. Also, the timer should count down correctly and trigger the lose condition if time runs out.
Finally, I'll make sure the text displays update correctly and the win or lose messages appear as needed. The game should reset or provide feedback when the game ends, but the user didn't specify, so maybe just showing the message is sufficient for now.
Overall, the approach is to keep it simple, use efficient methods, and ensure all features are implemented as per the user's request.
</think>
Here's a minimal web-based RPG game that meets your requirements:
This game includes:
- A top-down view with pixel graphics
- WASD controls for movement
- Trees that can be collected by walking into them
- A wood counter
- A 30-second countdown timer
- Win condition when player collects 10 pieces of wood
- Lose condition when time runs out
- Victory and defeat messages
- Simple collision detection
- Minimal graphics using circles to represent the player and trees
To play:
- Use WASD keys to move
- Walk into trees to collect wood
- Try to collect 10 pieces of wood before time runs out
- The game will end either in victory or defeat
- The current wood count and timer are displayed in the top-left corner
The game uses HTML5 Canvas for rendering and JavaScript for game logic. The graphics are intentionally kept minimal with circles representing both the player and trees.
🫧
Dot-com (2000): CAPE 44, Market Cap/GDP 150%
Financial Crisis (2008): CAPE 27, Market Cap/GDP 140%
Current (2025): CAPE > 37, Market Cap/GDP > 200%
Signing Ceremony ✍️
This moment is beyond epic. Tears were shared.
Why aren't we all just a product of holes
Recursive holes in space sounds fun.
spacetime curves into >= hole >= singularity =< hole <= out from curves spacetime
simultaneously. But from our perspective 'our' universe is expanding.
Wish I knew cool maths to go deep in quantum physics.
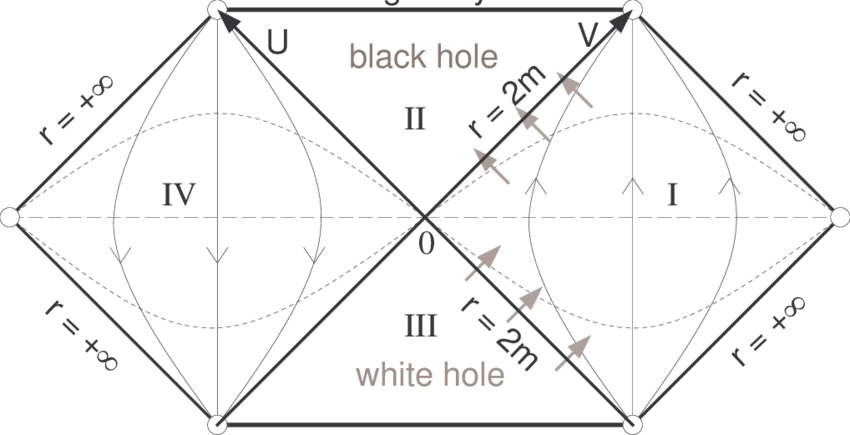
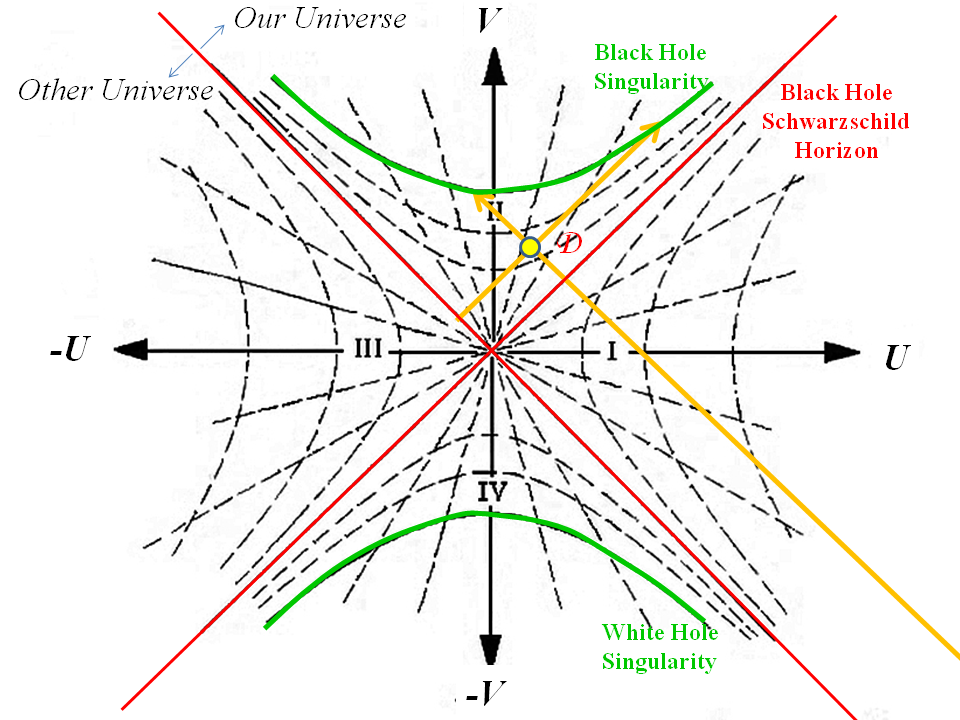
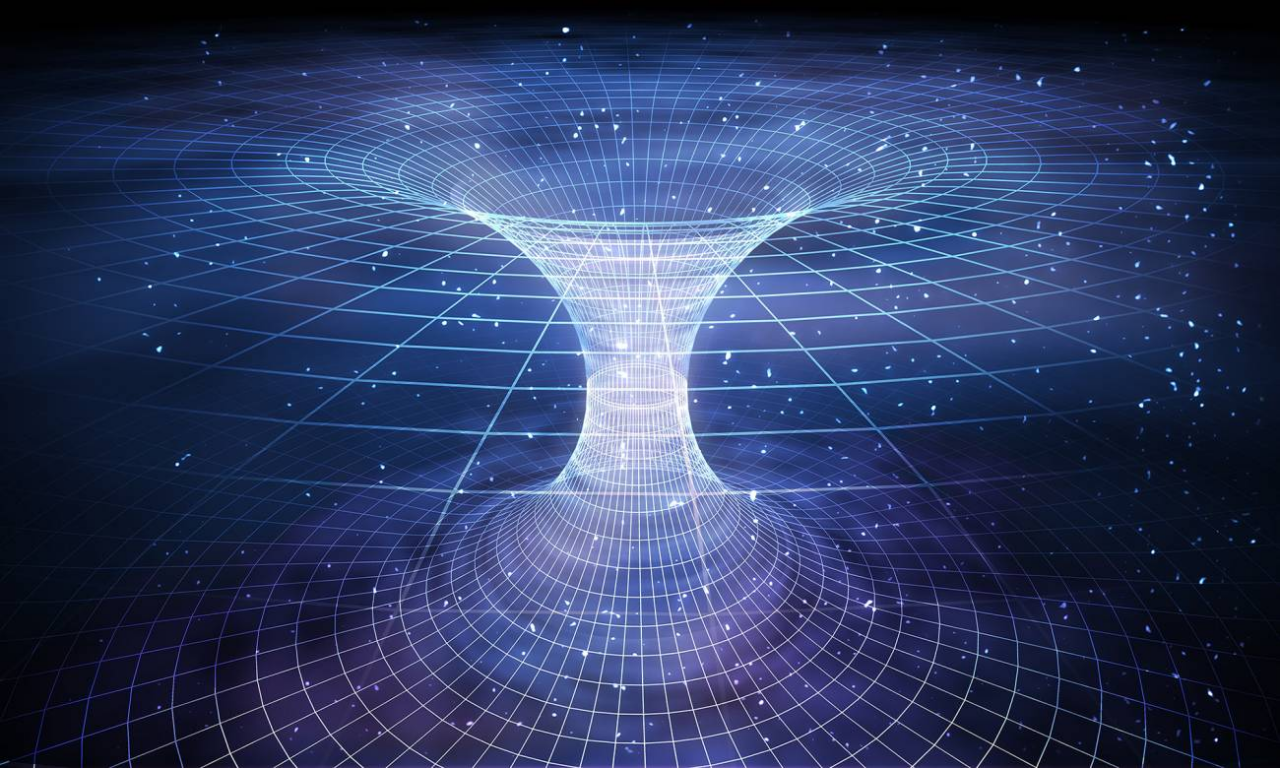
Yes, this is absolutely what I spend my time on at a Saturday evening.
Hmmm, LQG, AdS/CFT, ER=EPR, spin networks, causal dynamical triangulations...
Good thing I have Claude to ... er, I mean a beard to scratch and ponder this myself. Nah.. AI is great compared to classical information searching.
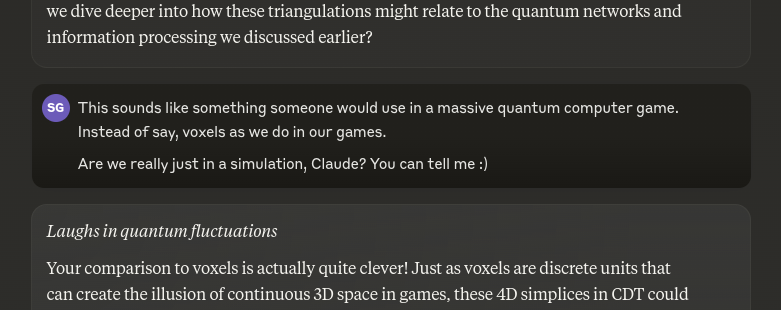
Happy caturday 🖖
|0⟩ --H-- (measurement)
Edit 2025-02-05; Oh my, just found our Veritasium has a video on this: